1. Tables
2. Select Statements
Select statements are used to get data from the database.
2.2. System variables
Get the current User:
select user from dual;
Name and Number of the row:
select rownum, name from players;
2.4. Alphanumerical Functions
Expressions | Description |
---|---|
length() |
gives the length from a String |
decode() |
allows you to replace certain parts of a String with another String doc |
substr() |
gives part of a given String doc |
instr() |
to find a certain part of a String in a given String doc |
upper() |
Transforms a given String to uppercase |
lower() |
Transforms a given String to lowercase |
2.5. Date Functions
Difference between 2 Dates:
select sysdate - pen_date from penalties;
Parse Date:
select to_date('2022-01-25', 'YYYY-MM-DD') from dual;
Date to String:
select to_char(sysdate, 'DD-MM-YY') from dual;
2.5.1. Formats for Dates
Examples:
select to_char(PEN_DATE, 'DD-MM.YY') from PENALTIES;
select to_char(sysdate, 'DD-MM.YY') from dual;
Formats:
FormatString | Description |
---|---|
DD, Dy, Day |
Days |
MM, Mon, Month |
Months (3 letters), (English Months) |
YY, YYYY |
Years (2 or 4 digits) |
HH, HH12, HH24 |
Hours (12 or 24h format) |
MI |
Minutes |
SS |
Seconds |
The case of the Formats will translate to the output |
2.6. Statistical Functions
Function | Description |
---|---|
count() |
counts the numer of rows |
min() |
gets the tiniest Value out of a column |
max() |
gets the biggest Value out of a column |
avg() |
average out of all the values |
stddev() |
Standard definition out of all the values |
variance() |
Variance between all the values |
Examples:
select count(*) from dual; /* returns: 1*/
select * from dual;
/* Amount of players that are from Stratford */
select count(*) from players where TOWN = 'Stratford';
/* highest penalty */
select max(AMOUNT) from PENALTIES;
3. Clauses
A SQL statement is made out of Clauses, and they could look like this;
select .. from ..
[WHERE ..]
[CONNECT BY ..]
[GROUP BY ..]
[HAVING ..]
[ORDER BY ..]
4. NULL
Null is special operator.
If a Value is NULL it is unknown or undefined.
if you want to check if a Value is null, you can do this with "is null"
You can not check null with "= null"! |
Given Value | Condition | Evaluates to: |
---|---|---|
10 |
is null |
false |
10 |
is not null |
true |
null |
is null |
true |
null |
is not null |
false |
10 |
!= null |
UNKNOWN |
10 |
= null |
UNKNOWN |
5. IN Operator
with the In operator, you can check if a value is in a list of Values.
Examples:
/* Players with the numbers 6, 8, 27, 44 and 104 */
select * from PLAYERS where PLAYERNO in (6, 8, 27, 44, 104)
/* with the help of subqueries:
All Players that have one or more Penalty: */
select * from PLAYERS
where PLAYERNO in (select distinct PLAYERNO from PENALTIES);
Example:
Output PlayerNo, name and initials of all Players, that have won at least 1 Match.
select PLAYERNO, NAME, INITIALS from PLAYERS
where PLAYERNO in
(select PLAYERNO from MATCHES where WON >= 1);
6. Order by
Order by sorts the output by a given value.
Example: All Towns and how many Players are in there sorted by Town
select town, NAME as Players from PLAYERS order by TOWN;
7. Group By
Groups the rows by a certain argument. For example groups the players by town:
select TOWN, count(*) as Anzahl
from PLAYERS
group by TOWN;
Amount of penalties for each year:
select to_char(PEN_DATE, 'yyyy') as YEAR, count(*) as AMOUNT, sum(AMOUNT) || ' €' as PENALTIES
from PENALTIES
group by to_char(PEN_DATE, 'yyyy')
order by 1;
8. JOIN
A select statement is a join, when there are at least 2 Tables and a where statement that connects the Tables with each other.
select * from PLAYERS, PENALTIES;
In this case we would get a cartesisches Product, that means that each row will be outputted with each row.
This is why we have to check that the Players and the penalties have the same Playerno.
select pl.PLAYERNO, pl.NAME, sum(pe.AMOUNT) as PENALTIES
from PLAYERS pl, PENALTIES pe
where pl.PLAYERNO = pe.PLAYERNO
group by pl.NAME, pl.PLAYERNO;
The most used Jointype is the Equijoin (Natural Join).
Join Types: Join_NEU.pdf
8.1. Natural Join
select emp.ENAME, dept.DNAME from EMP, DEPT
where EMP.DEPTNO = DEPT.DEPTNO;
or
select d.DEPTNO, e.ENAME, d.DNAME from EMP e, DEPT d
where e.DEPTNO = d.DEPTNO;
or
select EMP.ENAME, DEPT.DNAME from EMP NATURAL join DEPT;
/* it can be also be written as: */
select EMP.ENAME, DEPT.DNAME from EMP INNER join DEPT on EMP.DEPTNO = DEPT.DEPTNO;
inner Join combines the data from both Tables:
select * from EMP e inner join DEPT d on e.DEPTNO = d.DEPTNO;
8.2. Mengen
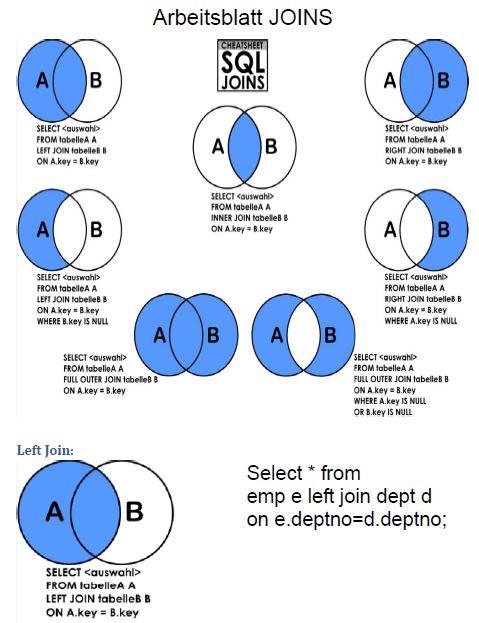
8.2.1. left join:
select *
from EMP e left join DEPT d
on e.DEPTNO = d.DEPTNO;
The outcome should be 15 rows long
8.2.2. inner join:
select *
from EMP e inner join DEPT d
on e.DEPTNO = d.DEPTNO;
The outcome should be 14 lines long, since the BIGBOSS does not have a DeptNo.
8.2.3. left join + null
select *
from EMP e left join DEPT d
on e.DEPTNO = d.DEPTNO
where e.DEPTNO is null or d.DEPTNO is null;
8.2.5. full outer join + null
select *
from EMP e full outer join DEPT d
on e.DEPTNO = d.DEPTNO
where d.DEPTNO is null or e.DEPTNO is null;
/* FIXME: Nächstes Mal*/
select *
from EMP e, DEPT d
where e.DEPTNO = d.DEPTNO (+) and (d.DEPTNO is null or e.DEPTNO is null);
8.3. Das Subquery (Innerselect)
Wieder ein select innerhalb der Bedingung.
-
keine Order by im Subquery
Suchreihenfolge:
1.Suche der Columns im Subquery
2. Wenn nicht vorhanden, Suche im Übergeordneten select
Trick: Alias-Namen |
Bsp:
Ausgabe von SpielerNr, Spielername derjenigen Spieler, die mindestens eine Strafe erhalten haben.
/* 1. Möglichkeit */
select distinct pl.PLAYERNO, pl.NAME
from PLAYERS pl inner join PENALTIES P
on pl.PLAYERNO = P.PLAYERNO
/* 2. Möglichkeit */
select PLAYERNO, NAME
from PLAYERS
where exists (
select * from PENALTIES
where PLAYERS.PLAYERNO = PENALTIES.PLAYERNO);
select PLAYERNO, NAME
from PLAYERS
where PLAYERNO in (select PLAYERNO from PENALTIES);
Bsp: Ausgabe der Spieler mit dne 4 höchsten Strafen
/* Gesamtsumme */
select *
from (
select pl.PLAYERNO, NAME, sum(P.AMOUNT) as AMOUNT
from PLAYERS pl inner join PENALTIES P
on pl.PLAYERNO = P.PLAYERNO
group by pl.PLAYERNO, pl.NAME
order by AMOUNT desc)
where ROWNUM <= 4;
/* Einzelne Strafen */
select * from (
select pl.PLAYERNO, pl.NAME, P.AMOUNT
from PLAYERS pl inner join PENALTIES P
on pl.PLAYERNO = P.PLAYERNO order by AMOUNT desc)
where ROWNUM <= 4;
9. Sessiondatum abändern
Einschub: Das Datumsformat für die Session kann jederzeit geändert werden, mithilfe folgendem Befehlt:
Dies änder das Datum nur für die aktuelle Session
alter session set nls_date_format = 'DD-MON-YYYY HH24:MI:SS';
10. Parts
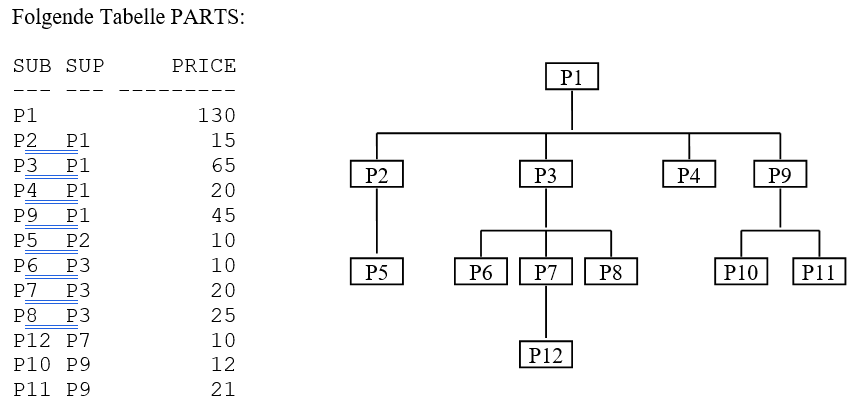
Hier kann man das UML Diagramm reverse-enginieeren

10.1. Parts.sql
neue SQL Datei: parts.sql
select *
from PARTS
connect by SUB = SUPER;
Bsp: Ermittle die Teile as denen P3 besteht:
select *
from PARTS
connect by SUB = SUPER
start with SUPER = 'P3';
Start With: Alle Bedingungen sind Möglich (SUPER < 3, …)
10.2. Unterschied zwischen START WITH (PRIOR) und WHERE:
Where entfernt nur die ihr entsprechenden Datensätze, keine Kind-DS
Systemvariable: LEVEL
Level is die Stufennummer beginnend mit 1
select rownum, level, SUB, SUPER, PRICE
from PARTS
connect by prior SUB = SUPER
start with SUPER = 'P3';
Skalarfunktion: LPAD
select lpad(' ', 8 * (level -1)) || level || '-' || SUPER || '-' || SUB
from PARTS
connect by prior SUB=SUPER
start with SUPER = 'P3';
11. Daten manipulieren
11.2. Ändern
UPDATE table_name SET column_name1 = expression | subquery
[, col_name2 = expression | subquery, ...]
[WHERE condition]
Where verwenden, sonst werden alle Zeilen geändert! |
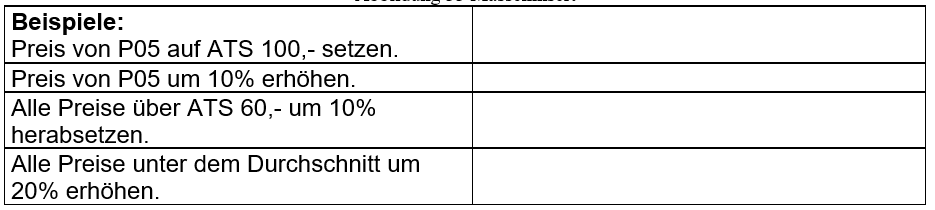
1: Preis von P05 auf ATS 100,- setzen
update PARTS
set PRICE = 100
where upper(SUB) = 'P5';
2: Preis von P05 um 10% erhöhen
update PARTS
set PRICE = PRICE * 1.1
where upper(SUB) = 'P5';
3: Alle Preise über ATS 60,- um 10% herabsetzen
update PARTS
set PRICE = PRICE * 0.9
where PRICE > 60;
4: Alle Preise unter dem Durchschnitt um 20% erhöhen.
update PARTS
set PRICE = PRICE * 1.2
where PRICE < (
select avg(PRICE)
from PARTS);
11.3. Löschen
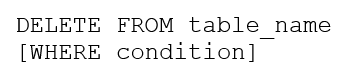
Löschbefehlte:
Befehl | Beschreibung |
---|---|
DELETE |
(DML) löscht nur die Daten, kann zurückgeholt werden |
DROP |
(DDL), alles wird gelöscht, kann nicht zurückgerollt werden |
TRUNCATE <TABLE> |
(DDL), gibt Speicherplatz frei, where nicht möglich, kann nicht zurückgerollt werden |
Aufgabe SQL 7 (15.03.2022, UNION, MINUS, INTERSECT, SUBSELECT, JOIN)
11.4. Nummerfolgen (Sequenzen)
Verwendet für PrimaryKey (künstlichen Schlüssen)
Variante: max
11.4.2. Variante 2: eigene Nummerntabelle
select max(TEAMNO) +1 from TEAMS;
insert into TEAMS values (..);
insert into TEAMNO values (...)
Beide Möglichkeiten haben Paralellsisierungsprobleme
11.4.3. Lösung: nicht automare statements
create sequence seq_name
[start with integer]
[increment by integer]
[{MAXVALUE integer | NOMAXVALUE}]
[{MINVALUE integer | NOMINVALUE}]
[{CYCLE | NOCYCLE}]
[{ORDER | NORODER}]
[{CACHE integer | NOCACHE}]
create sequence seq_teamno start with 3;
insert into TEAMS(teamsno, playerno, division)
values (seg_teamno.nextval, 104, 'first');
/* Values seg_teamno ausgeben: */
select seq_teamno.currval from dual;
Pseudospalten: nextval, curval
-
increment by ist default (1), auch negativmöglich
-
start with: Anfangswert
-
minvalue, maxvalue: mindest und höchstwert (limit erreicht bei ca. 10 ^ 27)
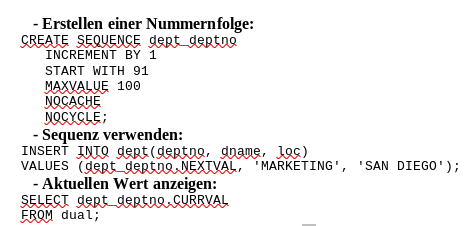
12. Integrity Rules (Constraints)
erzwingen Regeln
Rule | Bedeutung |
---|---|
NOT NULL |
can’t be null |
PK |
Primary key |
UNIQUE |
can’t exist multiple times |
FK |
Foreign key |
CHECK |
for more options |
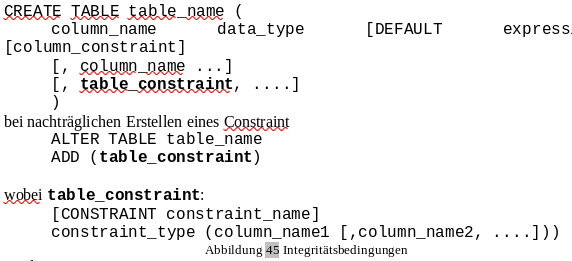
12.1. NOT NULL
column_name .... [CONSTRAINT constraint_name] NOT NULL
Name vom Constraints, wenn nicht angegeben, wir automatisch von ORACLE vergeben. (SYS_C[nnnn])
Wenn der Name vergeben wird, gibt es meist Datenbankregeln, die von der Firma festgelegt werden.
12.2. PK (PrimaryKey)
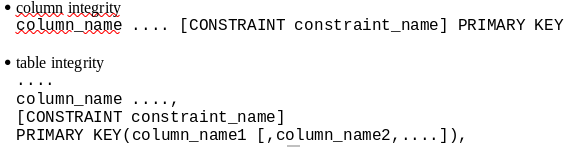
Der Primary key hat eigenschaften, die dieser gleich mitbringt:
-
not null
-
unique (darf einmal null sein)
column integrity nur möglich, wenn PK aus einer Spalte besteht.
Ein PK, der aus 2 Columns besteht, nennt man zusammengesetzter PK |
Beispiel column integrity:
create table teams (
teamno number(2) constraint pk_teams primary key,
playerno number(4),
division varchar2(6)
);
Beispiel table integrity:
create table teams (
teamno number(2),
playerno number(4),
division varchar2(6),
constraint pk_teams primary key(teamno)
);
12.3. UNIQUE
Unterschied zu PK:
-
Null hier möglich (1 mal)
-
mehrere UNIQUE Bedingungen pro Tabelle
Syntax:
-
column integrity:
column_name .... [CONSTRAINT constraint_name] REFERENCES table_name[(column_name1[,column_name2,....])] [ON DELETE CASCADE]
-
table integrity:
column_name ...., [CONSTRAINT constraint_name] UNIQUE (column_name1 [,column_name2,....]),
12.4. FK (Foreign Key)
On Delete Cascade, so werden die Sätze mit dem entsprechenden Fremdschlüsselwert automatisch mitgelöscht.
DML → Data Manipulation Language (update, update, delete)
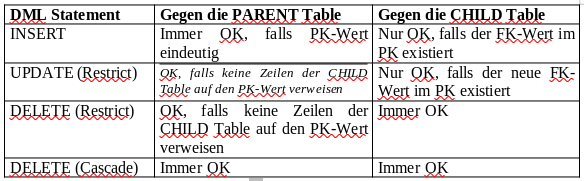
Tables in tennis-tables.sql.
13. TestStoff
-
ERD + RM
-
Create tables vom ERD (mit primary and foreign keys)
-
select statement
-
insert
-
update
-
alter
-
delete
-
drop
-
Sequenzes
-
Constraints (Primary key, foreign key)
13.1. Testbesprechung
→ SQL Developer (Verbindungsdaten auswendig lernen) → script zum Einspielen
-
Befehlt für Anzeigen von Tables:
select * from cat
/* oder */
select table_name from user_tables
-
Date format abändern:
alter session set nls_date_format = 'DD-MON-YYYY HH24:MI:SS';
-
distinct anwenden können
-
group by
-
joins!!!
-
concat → ||
-
like
-
to_date
-
substr
-
trim
-
upper, lower
-
nvl
-
to_char
-
to_date
-
order by
-
case:
SELECT cust_last_name,
CASE credit_limit WHEN 100 THEN 'Low'
WHEN 5000 THEN 'High'
ELSE 'Medium' END
FROM customers;
-
connect by (kommt nicht)
-
intersect (kommt nicht)
-
minus (kommt nicht)
-
sequences
-
subselect
-
update
-
insert
-
delete
-
drop
Die Tendenz auf dem Test liegt eher auf den select statements
Es gibt wieder die outputs
14. Index
Ein Index ist ein Attributwert + Adresse (Inhaltsverzeichnis)
Vorteil: kleiner daher leichter im Hauptspeicher platz findet
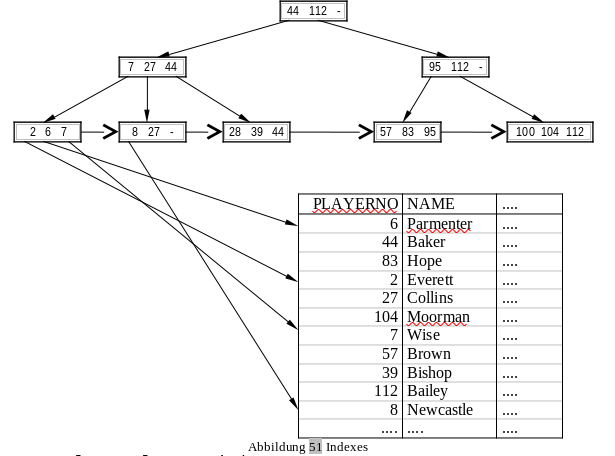
bei Oracle werden Indexe automatisch bei PK angelegt

DESCRIBE user_indexes;
select * from user_indexes;
select * from user_ind_columns;
Vorteil: select, update, delete
Nachteil: insert, update, delete
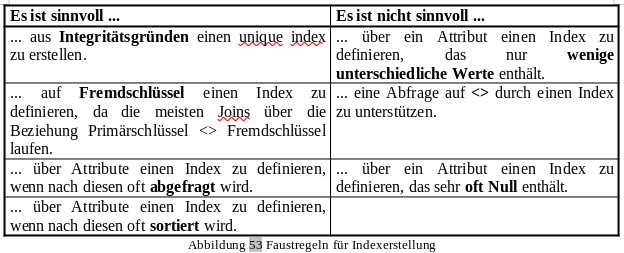
kein Index: (Faustregeln)
-
kleine Tabellen (wenig Zeilen)
-
Tabelle häufig aktualisiert
-
Abfragen rufen mehr als 2 - 4 % der Zeilen auf
-
Spalten werden nicht oft als Bedingungen verwendet
Beispiel:
create index pen_plno on PENALTIES (PLAYERNO);